Basically, a tuple (Tuple in C#) is an ordered sequence, immutable, fixed-size and of heterogeneous objects, ie, each object being of a specific type.
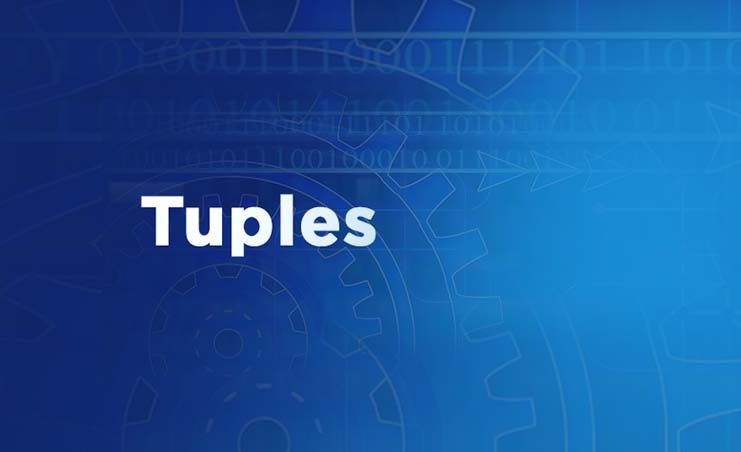
The tuples are not new in programming. They are already used in F#, Python and databases. However they are new to C#. The tuples were introduced in C# 4.0 with dynamic programming.
To learn more, visit: http://msdn.microsoft.com/en-us/library/system.tuple.aspx
Definitions of Tuples
“Each line that consists of an ordered list of columns is a record or tuple. The records may not contain information on all columns, and may take null values when this becomes necessary.”
http://pt.wikipedia.org/wiki/Banco_de_dados_relacional#Registros_.28ou_tuples.29
Example:
Insert Into Tb_clients values (1,’Frederico’, ‘1975-03-24’)
In this example,
(1,’Frederico’, ‘1975-03-24’)
is a tuple.
“A tuple, in mathematic, is a fixed-size ordered sequence of objects” http://es.wikipedia.org/wiki/Tuple
Example: In the equation 2×2 – 4x – 3, the sequence (2, -4, -3) is a tuple.
“An enupla (also known as n-tuple) is an ordered sequence of n elements, which can be defined by the ordered pair of recursion.
The main properties that distinguish an enupla are:
– An enupla can contain an object more than once.
– Objects are necessarily represented in the given order. ”
http://pt.wikipedia.org/wiki/Tuple
Tuples in .Net 4.0
While anonymous types have similar functionality in C#, they can not be used as return of methods, as can the type Tuple.
The KeyValuePair
A tuple is an ordered sequence, immutable, fixed size of heterogeneous objects.
Ordered sequence:
The order of items in a tuple follows the order used at the time of its creation.
Immutable:
All properties are read-only tuple, ie, once created, it can not be changed.
Fixed Size:
The size is set at the time of its creation. If it was created with three items, you can not add new items.
Of heterogeneous objects:
Each item has a specific and independent of the type of the other item.
Disadvantages:
As Tuples don’t have an explicit semantic meaning, your code becomes unreadable.
Creating Tuples:
In C#, Tuple is a static class that implements the “Factory” Pattern to create instances of Tuples. We can create an instance of a Tuple using the constructor or the static method “Create”.
The static method “Create” that returns an instance of type Tuple has eight overloads:

Example:

Tuples have a limit of 8 items. If you want to create a tuple with more items, we have to create nested Tuples.
The eighth item of the tuple has necessarily to be another Tuple. The example below will generate an exception.

To create a tuple with 8 items, we must do the following:

To create a tuple with more than 8 items, we do as follows:

What is a Tuple?
Tuples do not have names that may have some significance. The attributes of a tuple are called “Item1”, “Item2” and so on.
Two Tuples can be equal, but that doesn’t mean they are the same. Its meaning is not explicit, which can make your code less readable. For example, the following two tuples are equal, but represent different things:
(3, 9): Product Code 3 and Quantity 9
(3, 9): 3 and 9 are the codes of clients returned by a query.
As seen above, the fact that a tuple doesn’t carry information about its meaning, its use is generic and the developer decides what it will mean at the time of its creation and use.
So, why to use them?
A) Return of methods
Tuples provide a quick way to group multiple values into a single result, which can be very useful when used as a return of function, without the need to create parameters “ref” and / or “out “.
Example:

Another example of methods return is when we must return a list of an anonymous type. In this case we can easily replace this type by tuples.
Example:


C) Replace classes or structs that are created just to carry a return or to fill a list
Due to the interface IEquatable defines GetHashCode(), the implementation of the interface IStructuralEquatable creates a Hash code combining the members Hash codes, allowing the use of tuples as a composite key for a collection of type Dictionary.
Example:


B) Composite key in a Dictionary
Using the Tuple, we don’t need to create classes or structures to store only temporary values, such as creating a struct or class to add values to a combobox or listbox. With the tuples, it will no longer be necessary to create them.
Example:



Comparing and ordering
The interfaces IStructuralComparable and IStructuralEquatable were introduced in .Net 4.0 to assist in supporting Tuples.
A tuple is equal to another if and only if all items are equal, ie, t1.Item1 ==t2.Item1 and t1.Item2 == t2.Item2, and so on.
To sort, a comparison is made on individual items, ie, the comparison is made in the first Item1 if t1.Item1> t2.Item1 then Tuple t2 is the smallest, if t1.Item1 == t2.Item1 then the comparison is made in item2 and so on.
To use the interfaces IComparable, IEquatable, IStructuralComparable and IStructuralEquatable we must make the cast to the desired interface explicitly .

Conclusion
While the indiscriminate use of Tuples affect the readability of the code, its use at the appropriate time can be very handy for developers, allowing them to return multiple values from a function without the need to create parameters “ref” and / or “out “, allowing the creation of composite keys to collections of type Dictionary and eliminates the need to create structs or classes or just to fill combobox or lists.